The importance of testing JavaScript code.
As developers, it is imperative that we test our code not only once, but several times, in this age of agile development and continuous integration. Tests are an integral part of popular agile practices like test-driven development (TDD) and behavior-driven development (BDD).
If tests are so important, explain why they are so common in today's code. There are bugs in every software, and some of them may not become apparent until long after the program has been released. We reduce the likelihood that we will need to rewrite large programs by testing individual modules in as many potential configurations. Our time and money are saved by identifying and fixing defects early in the development cycle thanks to the tests we've put in place.
Having tests that document themselves is a wonderful benefit of TDD and BDD. Any team member may instantly grasp the purpose of a given block of code by reading through the associated tests.

How do you test JavaScript and what do you use?
Of course, you may use your preferred JS testing framework to build part of your tests from scratch. However, it won't take long until you become bored of repeating yourself with the same boilerplate code. Utilizing specialized tools is a considerably faster and more elegant approach to handling testing.
Identifying the different kinds of tests
Performance of a single method or class is evaluated during a unit test. Isolating a function is not easy since most programs are made up of interactions between modules. As we'll see in a moment, there are a few different methods to approach this problem using the JS test procedures. Unit tests are broken down even further into server-side (back end) and client-side (front end) varieties (on the front end).
We can replicate the whole user journey by calling on both the front and back ends of an application in what are called end-to-end (E2E) tests. E2E testing often involves automating user inputs such mouse clicks and keyboard strokes in a web browser to replicate real-world scenarios. Specialized test frameworks are designed to handle the complexities of end-to-end tests, which are the most difficult to execute throughout the testing process.
Finally, when used with unit tests, integration tests ensure that the system is working as intended. They are useful for verifying the interplay of various parts. All of the individual parts of an integration test may have passed their own unit tests, yet fail the overall test if they don't work together as planned. Such situations are seen in integration testing.
Describing the Test components
When doing unit tests, speed is of the highest concern. For the sake of efficiency, we'd rather not have to wait for a database to answer each time a minor function is tested. The unit tests should also be limited to the code being tested. Fortunately, there are defined standards within JavaScript testing that allow us to better isolate our applications and manage dependencies.
"Assertions" are the tests that determine whether or not a piece of code really produces the desired results. The assertions go unnoticed when our code works as expected. A developer will be made aware of a defect in the code when an assertion fails because of faulty code.
"Test doubles" (a pun on "stunt doubles") are used to manage external dependencies. To ensure that our system handles a given value correctly, we may create a "test double" by populating a database with known values. While there is a difference between mocks and stubs, they both serve the same objective of protecting your code by simulating real-world conditions.
There are times when we'd want to know more about the test we're taking. How many times, for instance, did a particular function really execute throughout the course of a test? "Spies" will be used for this purpose. The spy encapsulates the function and returns data about it, revealing the internal workings of our code.
When all the assertions, mocks, and spies have been set up, does that indicate the test pipeline is complete? Just barely. After all, you need to guarantee that every feature of your code has been thoroughly tested. Test coverage is a useful measure that determines how much of your code is really tested. How can you be sure that your software won't fail when a new condition is found if, for instance, your test only ever examines the if-condition of an if-else statement? While there is no magic solution for passing a test, it is helpful to do well on the test coverage section.
JavaScript Test Tools
Here, we provide a list of the most widely used JavaScript testing tools, organized by kind of test.
1.Client Side Unit Tests
After you have completed your unit and integration tests on the back end, you may choose to go on to testing the front-end components of your code. The front-end components of your application may be tested in different conditions with the aid of a test runner. Karma is widely used as a popular option for test runners. By default, it runs tests written in Mocha, Jasmine, and QUnit and was developed by the Angular JS team (another JavaScript unit testing framework).
2.Server-Side Unit and Integration Tests
JEST
A widely used testing framework is Jest. Facebook keeps it updated, and you can use it to try out other JavaScript frameworks like React, Angular, and Vue on it. This setup is substantially quicker than Mocha's since it includes mocking and assertion libraries. The speed with which tests may be performed is frequently cited as a strength of Jest by developers.
MOCHA
For applications written in Node.js, there is the Mocha testing framework. Mocha makes it simple to do asynchronous tests in JS (where one component must wait for another to send a command). Unlike some other frameworks, Mocha allows you to mix several assertion libraries, although this flexibility comes at the cost of a somewhat longer setup time.
CHAI
Unit testing both backend and frontend code is simplified using Chai, an assertion library designed specifically for the purpose. The system is compatible with any existing JavaScript testing framework.
JASMINE
Among the older frameworks, Jasmine stands out since it was created with the sole intention of supporting testing in behavior-driven development. Jasmine, like Jest, requires little configuration and provides convenient prebuilt features like test doubles and assertions. With Angular, this framework is the gold standard for testing.
3.End-to-End Tests
With end-to-end testing, you'll double-check the functionality of your apps by running them from both the server and the client's perspective. To do this, you may pretend to be a user and go through the process of doing something like creating an account or resetting a password. Testing from end to beginning, or E2E, has historically taken a considerable amount of time.
We are fortunate to have Cypress, an end-to-end testing framework created with developers in mind. Cypress can do its job quickly and with little effort from the user. We think so highly of Cypress as we discussed in a previous blog post, about Maintaining End-to-End Testing using Cypress with TestQuality, where we showed how one of our customer began building up test automation using Cypress once they had sketched out the manual tests they intended to run on a regular basis - roughly a hundred in total. Cypress is an excellent tool for performing end-to-end testing. It automatically records videos of test runs, and its test runner is Google Chrome, which almost all engineers are acquainted with. It also captures the activities it performed on the DOM on video, providing you with clear, visually debuggable recordings of failed test runs. It's a fantastic tool right out of the box.
Integrating Cypress Test Runs and Results with TestQuality
Advantages of using Cypress
- Extensive development experience! Running and debugging tests in the app is really convenient.
- Outstanding documentation! Cypress offers the nicest documentation you may imagine. During the installation and creation of the initial tests, our client just used documentation and examples from it.
- All in one instrument. Cypress includes a test runner, assertion library, HTTP request library, and many more features. There is no need to select several tools for developing testing infrastructure. It also allows plugins for additional functionality.
- Chai as a library of assertions (unlike Playwright with humble Jest expects).
- Getting elements and interactions with them include a built-in "wait till" for the element's actionable state.
- Access to network activities is simple. There is no need for external proxies to stub network requests and responses because it is built into Cypress.
- Custom command support is ready to use (and even types for you custom commands if you use TypeScript).
- Making screenshots on failures automatically.
Cypress Disadvantages
- Cypress has a large number of dependencies. Your node modules directory will become several tens of pounds heavier.
- It is not permitted to debug using console.log. You must use cy.log, which only requires a string.
- During the test run, all of the tests are somehow combined. This implies that variables with the same names cannot be used in various test files.
- Cypress did not start the first time I ran CI.
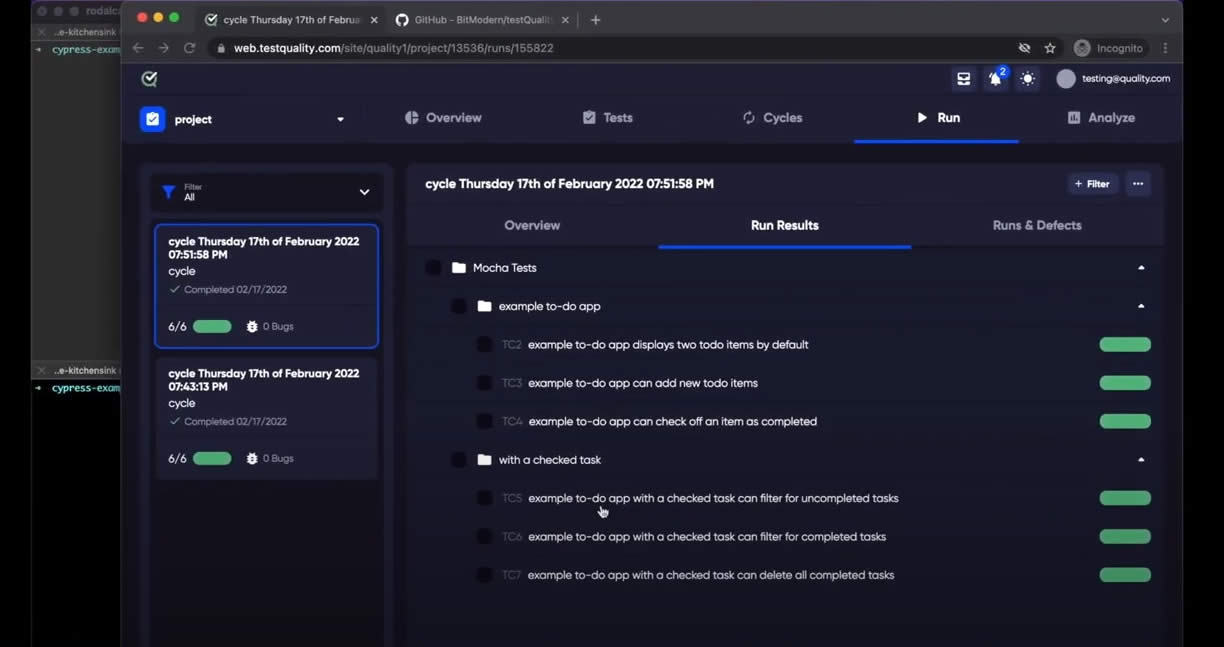
Cypress Test Run using a GitHub Cypress Test examples repository
How can I determine which tools are ideal for testing JavaScript?
The availability of suitable test frameworks is sometimes limited by the specifics of your setup and use case. For example, if you're a developer interested in doing end-to-end tests, Cypress is the way to go. In contrast, a server-side unit testing framework, like Mocha or Jest, is what you need to put your Node.js apps through their paces.Sometimes the framework you choose will determine what additional resources you have access to. Common combinations of Mocha with other tools for managing test flows include the Chai assertion library and the Sinon mocking tool.
If you choose Cypress because of the benefits and advantages mentioned above and also, it can be love at first sight, when you first start using Cypress and discover that integrating TestQuality with Jira or GitHub also works wonderfully in CI with little configuration!
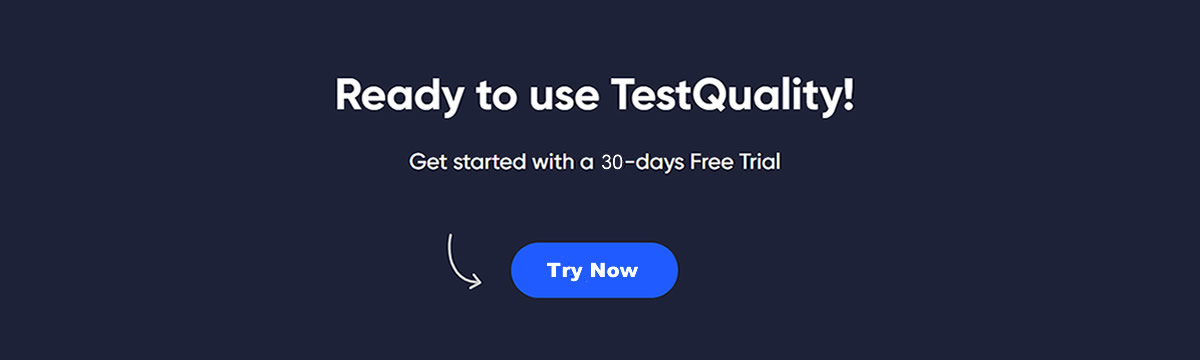